ArcPy Describe <dot> Properties
A tremendous amount of information is available through arcpy.Describe
. Until ArcGIS Pro 2.8 though, figuring out what properties were available for the object you had just described required digging into the documentation pretty deep.
Now, it is much easier to access these properties introspectively as a dictionary. Since I work so much in Jupyter, I love having the ability to have these also available as properties of an object, and also the very intuitive rendering of these properties as a Pandas DataFrame. Hence, I tossed this into a simple object.
import arcpy.da
from pathlib import Path
from typing import Union
class Describe:
def __init__(self, feature_layer):
# handle paths
aoi_fc = str(feature_layer) if isinstance(feature_layer, Path) else feature_layer
# map properties to object properties
self.__dict__ = arcpy.da.Describe(aoi_fc)
# toss it into a datframe for useful output
self.dataframe = pd.DataFrame.from_dict(self.__dict__, orient='index', columns=['value'])
self.dataframe.index.name = 'property'
def _repr_html_(self)->pd.DataFrame:
"""Acceses dataframe rendering for display in Jupyter"""
return self.dataframe._repr_html_()
True, there is overhead to creating a Pandas DataFrame, but my theory is if efficiency is the goal, you likely do not want this object anyway. Rather, I like this for the ease of introspection when trying to figure out what property I should be using.
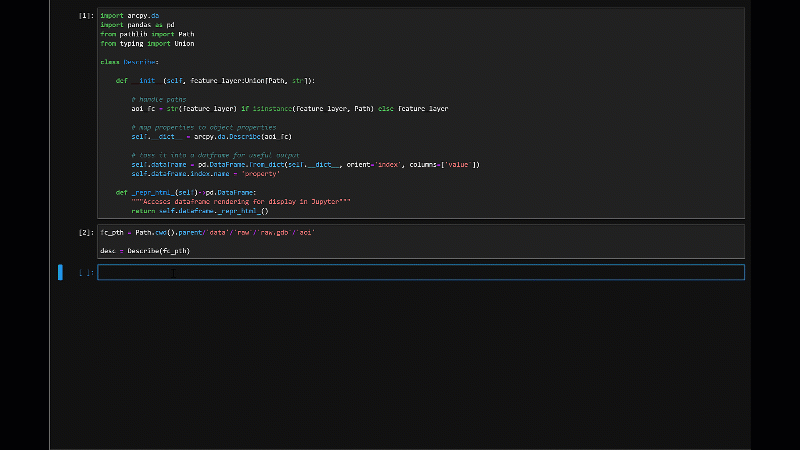
Have fun with this, and #GoPro!